[Algorithm] Array production problem
2024-09-26 17:36:51
Given an array of integers, return a new array such that each element at index i
of the new array is the product of all the numbers in the original array except the one at i
.
For example, if our input was [1, 2, 3, 4, 5]
, the expected output would be [120, 60, 40, 30, 24]
. If our input was [3, 2, 1]
, the expected output would be [2, 3, 6]
.
For example [1,2,3,4,5]:
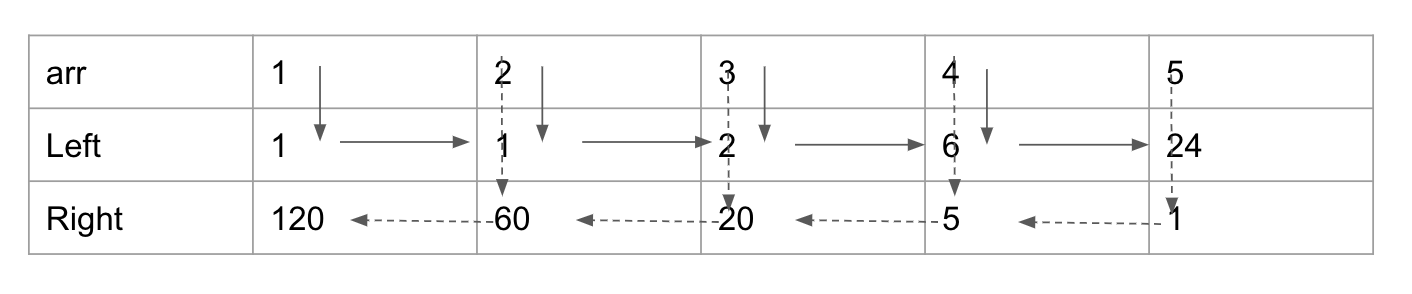
function productArr(arr) {
let left = [];
let right = [];
let prod = [];
left[0] = 1;
right[arr.length - 1] = 1; for (let i = 1; i <= arr.length -1; i++) {
left[i] = left[i-1] * arr[i-1];
} for (let j = arr.length - 2; j >=0; j--) {
right[j] = right[j+1] * arr[j+1];
} prod = left.map((l, i) => l * right[i]); return prod
} console.log(productArr([1, 2, 3, 4, 5])); // [120, 60, 40, 30, 24]
最新文章
- C#的HTTP协议中POST与GET的区别
- Java的List排序
- 自定义Windows性能监视器
- Bootstrap 我的学习记录2 栅格系统初识
- Sublime Text 下配置python
- 利用GBDT模型构造新特征具体方法
- hdoj 1106 排序
- linux中cat more less head tail 命令区别
- 从零开始制作jffs2文件系统
- 第六周O题(等边三角形个数)
- 教务处sso设计缺陷
- 利用commons-io.jar包中FileUtils和IOUtils工具类操作流及文件
- UILabel的讲解
- (NO.00001)iOS游戏SpeedBoy Lite成形记(二十五)
- Android NDK开发method GetStringUTFChars’could not be resolved
- Python的应用小案例
- golang goroutine
- random 模块 时间模块(time) sys模块 os模块
- Try Before Choosing
- MYSQL的用户变量(@)和系统变量(@@)
热门文章
- NOIP2012 D2 T2借教室
- 虚拟环境中pip install requirments.txt: Cannot fetch index base URL https://pypi.python.org/simple/
- ActiveMQ 权限(一)
- java 继承 String类
- CodeForces 602C The Two Routes(最短路)
- codevs 5294 挖地雷
- [UOJ30]/[CF487E]Tourists
- spoj 375 query on a tree LCT
- 使用SQL语句将数据库中的两个表合并成一张表
- ACM -- 算法小结(五)字符串算法之Sunday算法