Buffered Channels and Worker Pools
2024-10-21 07:26:58
原文链接:https://golangbot.com/buffered-channels-worker-pools/
buffered channels
- 带有缓冲区的channel 只有在缓冲区满之后 channel才会阻塞
WaitGroup
- 如果有多个 goroutine在后台执行 那么需要在主线程中 多次等待 可以有一个简单的方法 就是 通过WaitGroup 可以控制 Goroutines 直到它们都执行完成
例子
import (
"fmt"
"sync"
"time"
)
func process(i int, wg *sync.WaitGroup) {
fmt.Println("started Goroutine ", i)
time.Sleep(2 * time.Second)
fmt.Printf("Goroutine %d ended\n", i)
wg.Done()
}
func main() {
no := 3
var wg sync.WaitGroup
for i := 0; i < no; i++ {
wg.Add(1)
go process(i, &wg)
}
wg.Wait()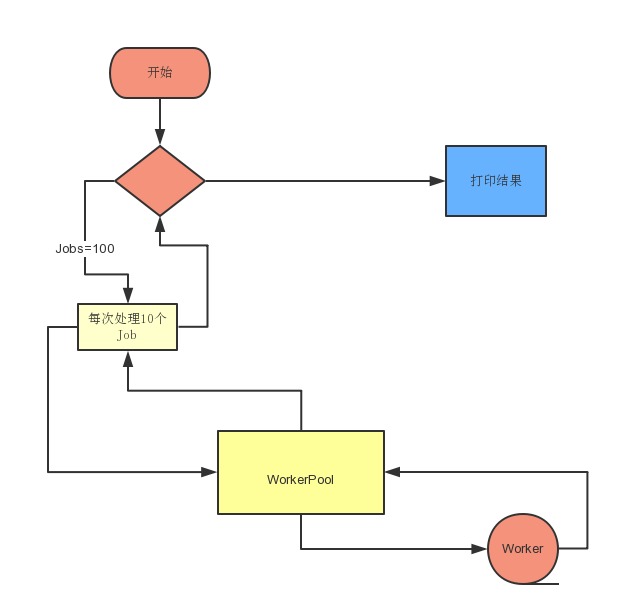
fmt.Println("All go routines finished executing")
}
Worker Pool Implementation
先贴一下个人理解的 程序执行的流程图
import (
"fmt"
"math/rand"
"sync"
"time"
)
type Job struct {
id int
randomno int
}
type Result struct {
job Job
sumofdigits int
}
var jobs = make(chan Job, 10)
var results = make(chan Result, 10)
func digits(number int) int {
sum := 0
no := number
for no != 0 {
digit := no % 10
sum += digit
no /= 10
}
time.Sleep(5 * time.Second)
return sum
}
func worker(wg *sync.WaitGroup) {
for job := range jobs {
output := Result{job, digits(job.randomno)}
results <- output
}
wg.Done()
}
func createWorkerPool(noOfWorkers int) {
var wg sync.WaitGroup
for i := 0; i < noOfWorkers; i++ {
wg.Add(1)
go worker(&wg)
}
wg.Wait()
close(results)
}
func allocate(noOfJobs int) {
for i := 0; i < noOfJobs; i++ {
randomno := rand.Intn(999)
job := Job{i, randomno}
jobs <- job
}
close(jobs)
}
func result(done chan bool) {
for result := range results {
fmt.Printf("Job id %d, input random no %d , sum of digits %d\n", result.job.id, result.job.randomno, result.sumofdigits)
}
done <- true
}
func main() {
startTime := time.Now()
noOfJobs := 100
go allocate(noOfJobs)
done := make(chan bool)
go result(done)
noOfWorkers := 10
createWorkerPool(noOfWorkers)
<-done
endTime := time.Now()
diff := endTime.Sub(startTime)
fmt.Println("total time taken ", diff.Seconds(), "seconds")
}
最新文章
- float4与half4数据类型
- Hummer框架平台介绍
- Dispatcher及线程操作
- java学习粗略路线
- 快速排序 javascript实现
- XML语法小结
- 五个数据段之代码段、数据段、BSS、栈、堆
- 类 Random
- World Finals 2017爆OJ记
- List Control控件
- WPS 2019 多个sheet表拆分成独立的excel文件
- 【Alpha版本】冲刺阶段——Day7
- ueditor 设置高度height. ue.setHeight(400); 设置宽度 width
- fabric-network_setup.sh安装脚本分析
- 【LOJ】#2532. 「CQOI2018」社交网络
- git学习(七):git 对象库
- php判断所在的客户端
- 【BZOJ 3136】 3136: [Baltic2013]brunhilda (数论?)
- FIS的安装
- linux文件操作篇 (一)文件属性与权限
热门文章
- Codeforces Round #431 (Div. 2) A
- Python基本的数据类型(补发)
- TaskFactory单例模式利用xml
- java exception ";file not found or file not exist";
- RabbitMQ使用教程(一)RabbitMQ环境安装配置及Hello World示例
- IIS服务器访问网站出现403错误的解决方法
- c#ADSL拨号类
- Java面向对象(static、final、匿名对象、内部类、包、修饰符、代码块)
- nodejs学习8:windows连接mongodb出现的错误解决办法
- class类型重定义,防止头文件重复加载