自定义 MessageBox 组件
2024-09-06 02:20:53
效果:
公共组件页面:
js部分:
<script>
export default {
props: {
title: {
type: String,
default: '标题'
},
content: {
type: String,
default: '这是弹框内容'
},
isShowInput: false,
inputValue: '',
isShowCancelBtn: {
type: Boolean,
default: true
},
isShowConfimrBtn: {
type: Boolean,
default: true
},
cancelBtnText: {
type: String,
default: '取消'
},
confirmBtnText: {
type: String,
default: '确定'
}
},
data () {
return {
isShowMessageBox: false,
resolve: '',
reject: '',
promise: '' // 保存promise对象
};
},
methods: {
// 确定,将promise断定为resolve状态
confirm: function () {
this.isShowMessageBox = false;
if (this.isShowInput) {
this.resolve(this.inputValue);
} else {
this.resolve('confirm');
}
this.remove();
},
// 取消,将promise断定为reject状态
cancel: function () {
this.isShowMessageBox = false;
this.reject('cancel');
this.remove();
},
// 弹出messageBox,并创建promise对象
showMsgBox: function () {
this.isShowMessageBox = true;
this.promise = new Promise((resolve, reject) => {
this.resolve = resolve;
this.reject = reject;
});
// 返回promise对象
return this.promise;
},
remove: function () {
setTimeout(() => {
this.destroy();
}, );
},
destroy: function () {
this.$destroy();
document.body.removeChild(this.$el);
}
}
};
</script>
css部分:
<style lang="less" scoped>
.message-box {
position: relative;
.mask {
position: fixed;
;
;
;
;
;
, , , 0.5);
}
.message-content {
position: fixed;
box-sizing: border-box;
padding: 1em;
min-width: 70%;
left: 50%;
top: 50%;
transform: translate(-50%, -50%);
border-radius: 0.4em;
background: #fff;
;
.icon {
position: absolute;
top: 1em;
right: 1em;
width: 0.9em;
height: 0.9em;
color: #878d99;
cursor: pointer;
&:hover {
color: #2d8cf0;
}
}
.title {
// font-size: 1.2em;
// font-weight: 600;
margin-bottom: 1em;
, , , );
font-size: 14px;
line-height: 150%;
font-weight: normal;
}
.content {
// font-size: 1em;
line-height: 2em;
// color: #555;
, , , );
font-size: 12px;
line-height: 150%;
}
input {
width: 100%;
;
background-color: #fff;
border-radius: 0.4em;
border: 1px solid #d8dce5;
box-sizing: border-box;
color: #5a5e66;
display: inline-block;
font-size: 14px;
height: 3em;
;
outline: none;
1em;
&:focus {
border-color: #2d8cf0;
}
}
.btn-group {
margin-top: 1em;
float: right;
overflow: hidden;
.btn-default {
padding: 0.8em 1.5em;
font-size: 1em;
color: #555;
border: 1px solid #d8dce5;
border-radius: 0.2em;
cursor: pointer;
background-color: #fff;
outline: none;
&:hover {
color: #2d8cf0;
border-color: #c6e2ff;
background-color: #ecf5ff;
}
}
.btn-primary {
padding: 0.8em 1.5em;
font-size: 1em;
color: #fff;
border-radius: 0.2em;
cursor: pointer;
border: 1px solid #2d8cf0;
background-color: #2d8cf0;
outline: none;
&:hover {
opacity: .8;
}
}
.btn-confirm {
margin-left: 1em;
}
}
}
}
.handle_btn{
position: relative;
bottom: -20px;
>ul{
display: flex;
justify-content: space-between;
text-align: center;
>li{
width: 45%;
span{
border-radius: 6px;
font-size: 14px;
line-height: 150%;
}
}
// >li:first-child{
// }
}
}
</style>
main全局:
公共的js设置全局属性
import msgboxVue from './index.vue';
// 定义插件对象
const MessageBox = {};
// vue的install方法,用于定义vue插件
MessageBox.install = function(Vue, options) {
const MessageBoxInstance = Vue.extend(msgboxVue);
let currentMsg;
const initInstance = () => {
// 实例化vue实例
currentMsg = new MessageBoxInstance();
let msgBoxEl = currentMsg.$mount().$el;
document.body.appendChild(msgBoxEl);
};
// 在Vue的原型上添加实例方法,以全局调用
Vue.prototype.$msgBox = {
showMsgBox(options) {
if (!currentMsg) {
initInstance();
}
if (typeof options === 'string') {
currentMsg.content = options;
} else if (typeof options === 'object') {
Object.assign(currentMsg, options);
}
return currentMsg.showMsgBox()
.then(val => {
currentMsg = null;
return Promise.resolve(val);
})
.catch(err => {
currentMsg = null;
return Promise.reject(err);
});
}
};
};
export default MessageBox;
<!-- 自定义 MessageBox 组件 -->
<template>
<div class="message-box" v-show="isShowMessageBox">
<div class="mask" @click="cancel"></div>
<div class="message-content">
<!-- <svg class="icon" aria-hidden="true" @click="cancel"> -->
<!-- <use xlink:href="#icon-delete"></use> -->
<!-- </svg> -->
<h3 class="title">{{ title }}</h3>
<p class="content">{{ content }}</p>
<div>
<input type="text" v-model="inputValue" v-if="isShowInput" ref="input" @keyup.enter="confirm">
</div>
<div class="handle_btn">
<ul>
<li @click="cancel" v-show="isShowCancelBtn"><span style="color: rgba(166, 166, 166, 1);">{{ cancelBtnText }}</span></li>
<li @click="confirm" v-show="isShowConfimrBtn"><span style="color: rgba(254, 100, 98, 1);">{{ confirmBtnText }}</span></li>
</ul>
</div>
<div class="btn-group">
<!-- <button class="btn-default" @click="cancel" v-show="isShowCancelBtn">{{ cancelBtnText }}</button> -->
<!-- <button class="btn-primary btn-confirm" @click="confirm" v-show="isShowConfimrBtn">{{ confirmBtnText }}</button> -->
</div>
</div>
</div>
</template>
调用:
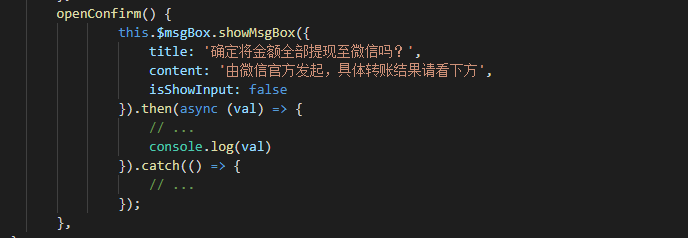
最新文章
- 写自己的Socket框架(一)
- 交换机做Channel-Group
- caffe中的props
- Swagger简介
- 用Firefly创建第一个工程
- 【模拟】NEERC15 A Adjustment Office (2015-2016 ACM-ICPC)(Codeforces GYM 100851)
- windows下搭建Apache+Mysql+PHP开发环境
- JJG 623-2005 电阻应变仪计量检定规程
- 学习RocketMQ (一) 安装并且启动MQ
- Microsoft Visual Studio 2017 编译最新版 libuv 1.x
- 原生js的联动全选
- xpath爬取新浪天气
- Vmware的虚拟机示例进入BIOS方法
- VIVE pro和hololens购买调研
- 自定义WPF控件(MyTextBox、MyDatePicker、MyDataGrid)
- 如何整合Office Web Apps至自己开发的系统(二)
- Java使用Itext5.5.10进行pdf签章
- Linux考前突击
- 关于";undefined reference";错误
- 给开发者准备的 10 款最好的 jQuery 日历插件[转]
热门文章
- lik模糊e查询语句,索引使用效果详解
- gitlab私钥配置
- 消费者与生产者---LinkedList方式模拟
- linux中设置虚拟域名
- 注解@requestBody自动封装复杂对象 (成功,自己的例子封装的不是一个复杂对象,只是一个简单的User对象,将jsp页面的name转成json字符串,再用JSON.stringify()传参就行了)
- Heartbeat安装及配置
- Arthas阿里开源的 Java 诊断工具
- Struts2基础-4 -struts拦截器
- Python 中内建属性 __getattribute__
- JS中数据结构之集合