POJ 2826 An Easy Problem?!(线段交点+简单计算)
2024-08-26 23:40:00
Description
It's raining outside. Farmer Johnson's bull Ben wants some rain to water his flowers. Ben nails two wooden boards on the wall of his barn. Shown in the pictures below, the two boards on the wall just look like two segments on the plane, as they have the same width.
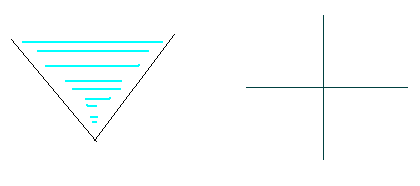
Your mission is to calculate how much rain these two boards can collect.
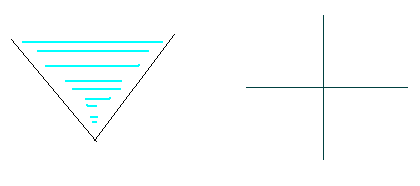
Your mission is to calculate how much rain these two boards can collect.
Input
The first line contains the number of test cases.
Each test case consists of 8 integers not exceeding 10,000 by absolute value, x1, y1, x2, y2, x3, y3, x4, y4. (x1, y1), (x2, y2) are the endpoints of one board, and (x3, y3), (x4, y4) are the endpoints of the other one.
Each test case consists of 8 integers not exceeding 10,000 by absolute value, x1, y1, x2, y2, x3, y3, x4, y4. (x1, y1), (x2, y2) are the endpoints of one board, and (x3, y3), (x4, y4) are the endpoints of the other one.
Output
For each test case output a single line containing a real number with precision up to two decimal places - the amount of rain collected.
题目大意:给两条线段,问这两条线段可以接多少面积的雨水。
思路:没有相交的线段不能接水。因为雨是垂直下落的,有时可能会有一条线段遮住了接水的地方导致无法接水。
PS:输出就不加EPS可能会WA,比如某个计算结果是0.004999999999……
代码(32MS):
#include <cstdio>
#include <cstring>
#include <iostream>
#include <algorithm>
#include <cmath>
using namespace std; const double EPS = 1e-;
const double PI = acos(-1.0);//3.14159265358979323846 inline int sgn(double x) {
return (x > EPS) - (x < -EPS);
} struct Point {
double x, y;
Point() {}
Point(double x, double y): x(x), y(y) {}
void read() {
scanf("%lf%lf", &x, &y);
}
bool operator < (const Point &rhs) const {
if(y != rhs.y) return y < rhs.y;
return x < rhs.x;
}
Point operator + (const Point &rhs) const {
return Point(x + rhs.x, y + rhs.y);
}
Point operator - (const Point &rhs) const {
return Point(x - rhs.x, y - rhs.y);
}
Point operator * (const int &b) const {
return Point(x * b, y * b);
}
Point operator / (const int &b) const {
return Point(x / b, y / b);
}
double length() const {
return sqrt(x * x + y * y);
}
Point unit() const {
return *this / length();
}
};
typedef Point Vector; double dist(const Point &a, const Point &b) {
return (a - b).length();
} double cross(const Point &a, const Point &b) {
return a.x * b.y - a.y * b.x;
}
//ret >= 0 means turn left
double cross(const Point &sp, const Point &ed, const Point &op) {
return sgn(cross(sp - op, ed - op));
} double area(const Point& a, const Point &b, const Point &c) {
return fabs(cross(a - c, b - c)) / ;
} struct Seg {
Point st, ed;
Seg() {}
Seg(Point st, Point ed): st(st), ed(ed) {}
void read() {
st.read(); ed.read();
}
};
typedef Seg Line; bool isIntersected(const Point &s1, const Point &e1, const Point &s2, const Point &e2) {
return (max(s1.x, e1.x) >= min(s2.x, e2.x)) &&
(max(s2.x, e2.x) >= min(s1.x, e1.x)) &&
(max(s1.y, e1.y) >= min(s2.y, e2.y)) &&
(max(s2.y, e2.y) >= min(s1.y, e1.y)) &&
(cross(s2, e1, s1) * cross(e1, e2, s1) >= ) &&
(cross(s1, e2, s2) * cross(e2, e1, s2) >= );
} bool isIntersected(const Seg &a, const Seg &b) {
return isIntersected(a.st, a.ed, b.st, b.ed);
} bool isParallel(const Seg &a, const Seg &b) {
return sgn(cross(a.ed - a.st, b.ed - b.st)) == ;
} //return Ax + By + C =0 's A, B, C
void Coefficient(const Line &L, double &A, double &B, double &C) {
A = L.ed.y - L.st.y;
B = L.st.x - L.ed.x;
C = L.ed.x * L.st.y - L.st.x * L.ed.y;
} Point intersection(const Line &a, const Line &b) {
double A1, B1, C1;
double A2, B2, C2;
Coefficient(a, A1, B1, C1);
Coefficient(b, A2, B2, C2);
Point I;
I.x = - (B2 * C1 - B1 * C2) / (A1 * B2 - A2 * B1);
I.y = (A2 * C1 - A1 * C2) / (A1 * B2 - A2 * B1);
return I;
} bool isEqual(const Line &a, const Line &b) {
double A1, B1, C1;
double A2, B2, C2;
Coefficient(a, A1, B1, C1);
Coefficient(b, A2, B2, C2);
return sgn(A1 * B2 - A2 * B1) == && sgn(A1 * C2 - A2 * C1) == && sgn(B1 * C2 - B2 * C1) == ;
} /*******************************************************************************************/ Seg a, b;
int n; double solve() {
if(!isIntersected(a, b)) return ;
Seg tmp(Point(, ), Point(, ));
if(isParallel(a, tmp) || isParallel(b, tmp) || isParallel(a, b)) return ;
if(a.st.y > a.ed.y) swap(a.st, a.ed);
if(b.st.y > b.ed.y) swap(b.st, b.ed);
Point O = intersection(a, b);
if(b.ed < a.ed) swap(a, b);
if(sgn(a.ed.x - O.x) == sgn(b.ed.x - O.x) && (sgn(b.ed.x - O.x) * sgn(cross(b.ed, O, a.ed)) >= ) &&
sgn(fabs(b.ed.x - O.x) - fabs(a.ed.x - O.x)) >= ) return ;
Point A = a.ed;
tmp = Seg(A, Point(A.x + , A.y));
Point B = intersection(tmp, b);
return area(A, B, O);
} int main() {
scanf("%d", &n);
for(int i = ; i < n; ++i) {
a.read(), b.read();
printf("%.2f\n", solve() + EPS);
}
}
最新文章
- Python的多类型传值和冗余参数
- 今天第一节PS课
- (转)ASP.NET Mvc 2.0 - 1. Areas的创建与执行
- Async 和 Await的性能(.NET4.5新异步编程模型)
- Android 的 ramdisk.img、system.img、userdata.img 作用说明,以及UBoot 系统启动过程
- SQL server 常见用法记录
- Xcode 合并分支报错
- iPhone开发 - 常用库
- Android 应用框架 —— 组件
- 百度ueditor 上传图片后如何设置样式
- onclick用法 超链接简单弹出窗口实例
- RTC定时开机闹钟
- JavaScript 堆
- JavaEE Tutorials (4) - 企业bean入门
- C#中DBNull.Value和Null的用法和区别
- MySQL 存储过程错误处理
- JavaScript case 条件语句
- solr实现动态加载分词
- jdk动态代理在idea的debug模式下不断刷新tostring方法
- MySQL查询where条件的顺序对查询效率的影响
热门文章
- iOS之改变UIAlertViewController字体的颜色
- Spring知识点小结(三)
- 键盘录入6个int类型的数据存入数组arr中,将arr数组中的内容反转...
- Const 关键字详解
- Systemd简介与使用
- 使用EF Core的CodeFirt 出现的问题The specified framework version &#39;2.1&#39; could not be parsed
- 如何分析Mysql慢SQL
- python学习笔记:第20天 多继承、MRO C3算法
- C# 获取UTC 转换时间戳为C#时间
- 全国Uber优步司机奖励政策 (1月4日-1月10日)